Unlock the potential of smarter applications by seamlessly integrating Java with OpenAI’s ChatGPT (GPT-3.5-turbo model) via the API. In this comprehensive guide, we’ll walk you through the process, providing clear explanations and example code to elevate your application’s user experience by using the ChatGPT API in your Java code. Let’s get started!
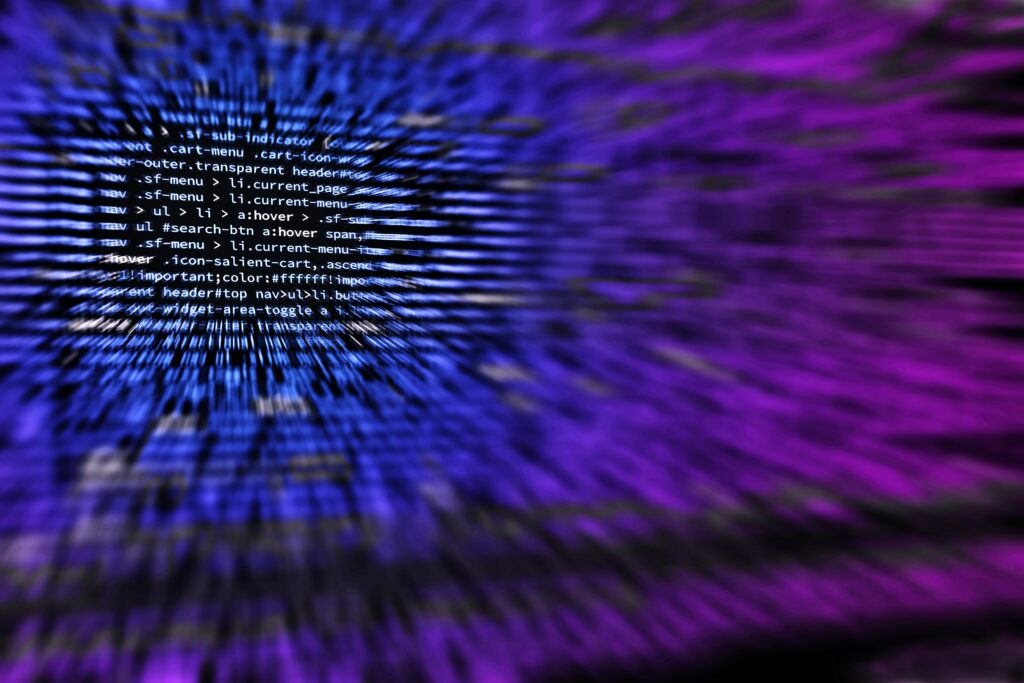
Step 1: Obtain Your OpenAI ChatGPT API Key
Before diving into the integration the ChatGPT API in Java, sign up on OpenAI’s platform to obtain your API key. This key serves as the authentication mechanism for your requests. Once registered, access your OpenAI dashboard. This is where you’ll find your API key and manage other settings related to your OpenAI account. Within your dashboard, navigate to the API section. Here, you’ll create your unique API key. This key is a confidential credential that should be handled with care, as it grants access to OpenAI’s powerful language model. Copy your API key to the clipboard. This key will be integrated into your Java code to authenticate requests to the ChatGPT API.
Understanding ChatGPT API Pricing
Embarking on the journey of integrating ChatGPT into your Java application requires a clear understanding of the associated costs. The pricing structure is simple and flexible, allowing you to pay only for the resources you utilize. Here’s a breakdown of the costs associated with various models:
- GPT-4 Turbo:
- gpt-4-0125-preview: $0.01 per 1K tokens (Input), $0.03 per 1K tokens (Output)
- gpt-4-1106-preview: $0.01 per 1K tokens (Input), $0.03 per 1K tokens (Output)
- GPT-4:
- gpt-4: $0.03 per 1K tokens (Input), $0.06 per 1K tokens (Output)
- gpt-4-32k: $0.06 per 1K tokens (Input), $0.12 per 1K tokens (Output)
- GPT-3.5 Turbo:
- gpt-3.5-turbo-0125: $0.0005 per 1K tokens (Input), $0.0015 per 1K tokens (Output)
- gpt-3.5-turbo-instruct: $0.0015 per 1K tokens (Input), $0.0020 per 1K tokens (Output)
Step 2: ChatGPT API Integration in Java
Now, let’s delve into the Java code to establish a connection with the OpenAI API and harness the power of ChatGPT.
Setting Up the Java Classes
Import the necessary Java classes for handling HTTP connections and I/O operations. Create a class named ChatGPT
, which will contain the integration code.
Defining the ChatGPT Method
Within the ChatGPTAPIExample
class, define the chatGPT
method. This method takes a user prompt as input and returns the response from ChatGPT. It handles the HTTP connection, sends requests to the ChatGPT API endpoint, and processes the JSON-formatted response.
Configuring Parameters
Specify essential parameters such as the API endpoint URL, your OpenAI API key obtained in Step 1, and the ChatGPT model you intend to use (e.g., gpt-3.5-turbo
).
Handling HTTP POST Request (API)
Create an HTTP POST request, open a connection, set up request headers, and define the request body using a JSON string that includes the model and the user prompt.
Processing the Response
Retrieve and process the response from ChatGPT. Extract the relevant content from the JSON format.
Main Method Execution
In the main
method, test the code by calling the chatGPT
method with a sample prompt. The response will be displayed in the console.
Example: ChatGPT API Integration with Java Code
import java.io.*; import java.net.HttpURLConnection; import java.net.URL; public class ChatGPT { public static String generateChatGPTResponse(String userPrompt) { String apiURL = "https://api.openai.com/v1/chat/completions"; String apiKey = "PASTE_YOUR_API_KEY"; String LLMname = "gpt-3.5-turbo"; try { // Create URL object URL url = new URL(apiURL); // Open connection HttpURLConnection connection = (HttpURLConnection) url.openConnection(); // Set the request method to POST connection.setRequestMethod("POST"); // Set request headers connection.setRequestProperty("Authorization", "Bearer " + apiKey); connection.setRequestProperty("Content-Type", "application/json"); // Create the request body String requestBody = "{\"model\": \"" + LLMname + "\", \"messages\": [{\"role\": \"user\", \"content\": \"" + userPrompt + "\"}]}"; // Enable input/output streams connection.setDoOutput(true); // Write the request body try (OutputStreamWriter writer = new OutputStreamWriter(connection.getOutputStream())) { writer.write(requestBody); writer.flush(); } // Read the response try (BufferedReader reader = new BufferedReader(new InputStreamReader(connection.getInputStream()))) { StringBuilder response = new StringBuilder(); String line; while ((line = reader.readLine()) != null) { response.append(line); } return getLLMResponse(response.toString()); } } catch (IOException e) { throw new RuntimeException("Error interacting with the ChatGPT API: " + e.getMessage(), e); } } private static String getLLMResponse(String response) { int firstChar = response.indexOf("content") + 11; int lastChar = response.indexOf("\"", firstChar); return response.substring(firstChar, lastChar); } public static void main(String[] args) { String userPrompt = "Hi robot, this is my first API call! I'm so excited! Tell me a joke!"; String chatGPTResponse = generateChatGPTResponse(userPrompt); System.out.println(chatGPTResponse); } }
Conclusion and Caution
Congratulations! You’ve successfully integrated ChatGPT API in Java using the OpenAI API. It’s crucial to note that while ChatGPT is a powerful tool, responses should be thoroughly vetted before deploying in production.
Note: Be aware of rate limits. If you encounter a 429 error („Too Many Requests“), consider creating a new OpenAI account or upgrading to a plan with extended rate limits for the API.